This is a simple health guard project on Colab. In the future, it can be enhanced with AI to analyze symptoms, identify possible diseases, and assess severity. Let’s move forward!
Step 1 : Install transformers pandas numpy scikit-learn
!pip install transformers pandas numpy scikit-learn
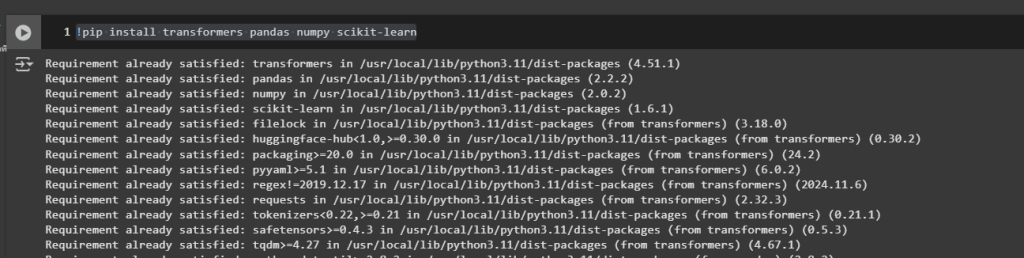
Step 2 : Create sample data (in practice, use real data from hospitals or reliable sources)
import pandas as pd
# Create sample data (in practice, use real data from hospitals or reliable sources)
data = {
"Symptoms": ["Headache, fever", "Right abdominal pain, fever", "Cough, sore throat", "Dizziness, nausea", "Itchy rash"],
"Possible Disease": ["Common cold", "Appendicitis", "Flu", "Food poisoning", "Allergy"],
"Recommendation": ["Rest and drink plenty of water", "See a doctor within 6 hours", "Take cough medicine and rest", "Drink electrolyte solution", "Take antihistamines"],
"Severity": ["Low", "High", "Moderate", "Moderate", "Low"]
}
df = pd.DataFrame(data)
df.to_csv("health_data.csv", index=False)

Step 3 : This step including load data, Convert message to feature, training, create model and evaluation model.
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
#load data
df = pd.read_csv("health_data.csv")
#Convert message to feature
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(df["Symptoms"])
y = df["Severity"]
#Train
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
#Create Model
model = RandomForestClassifier()
model.fit(X_train, y_train)
#Evaluation model
accuracy = model.score(X_test, y_test)
print(f"Accuracy: {accuracy:.2f}")
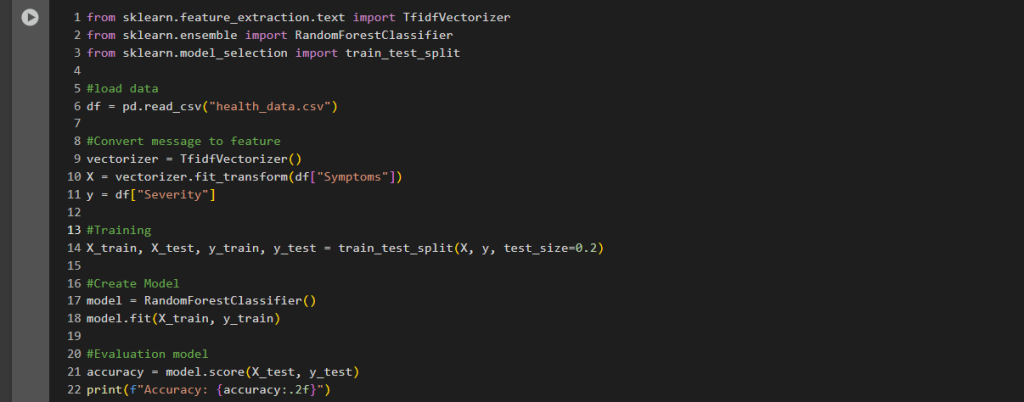
Step 4: Create assess_symptons function for prediction
def assess_symptoms(symptoms):
# Convert to feature
symptoms_vec = vectorizer.transform([symptoms])
# Prediction
prediction = model.predict(symptoms_vec)[0]
possible_conditions = df[df["Symptoms"].str.contains(symptoms.split()[0])]["Possible Disease"].values
advice = df[df["Symptoms"].str.contains(symptoms.split()[0])]["Recommendation"].values
return {
"Symptoms": symptoms,
"Possible Disease": list(set(possible_conditions))[:3],
"Recommendation": list(set(advice))[:3],
"Severity": prediction
}
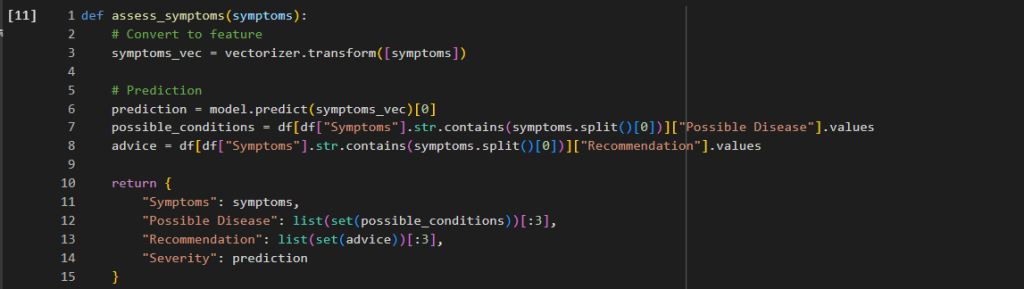
Step 5 : For test symptom Headache, fever
# Test
test_symptoms = "Headache, fever"
result = assess_symptoms(test_symptoms)
print("Evaluation results")
for key, value in result.items():
print(f"{key}: {value}")
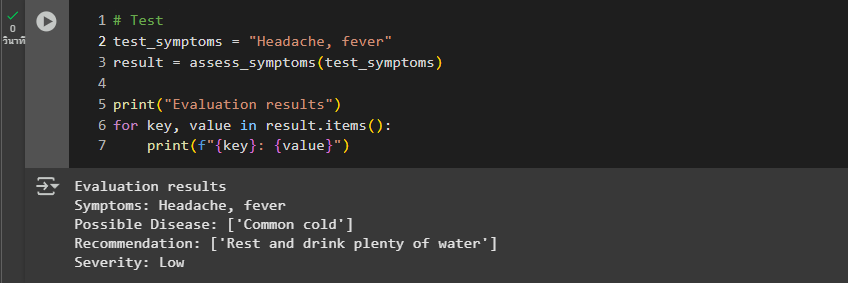
Step 6 : Using IPython display
from IPython.display import display
import ipywidgets as widgets
symptom_input = widgets.Text(
placeholder='Enter your symptoms (e.g. "headache, fever")',
description='Symptoms:',
disabled=False
)
output = widgets.Output()
def on_submit(b):
with output:
output.clear_output()
symptoms = symptom_input.value
if symptoms:
result = assess_symptoms(symptoms)
print("\nEvaluation results:")
for key, value in result.items():
print(f"{key}: {value}")
else:
print("Please enter your symptoms")
submit_button = widgets.Button(description="Assess symptoms")
submit_button.on_click(on_submit)
display(symptom_input, submit_button, output)
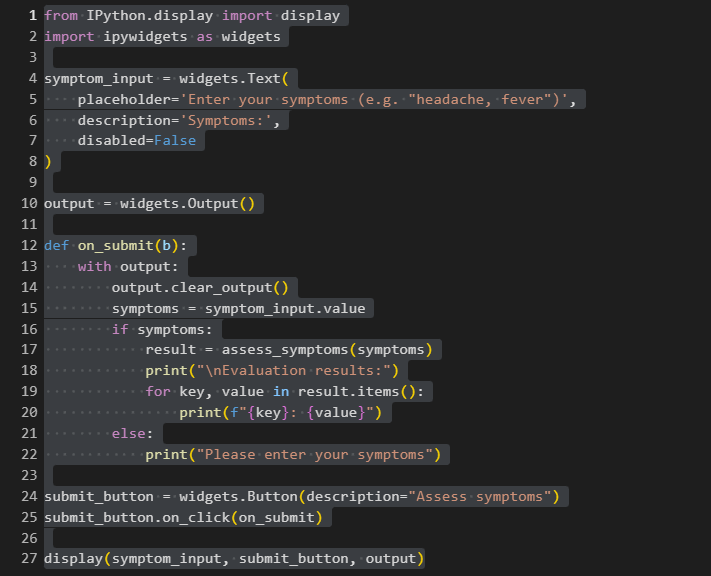
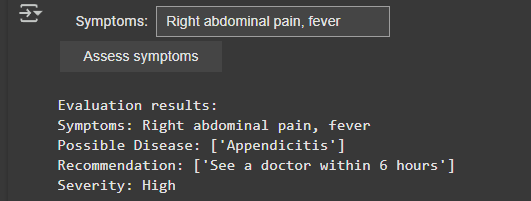
If you can develop a program that can help people recover from pain, I would be very happy. for source code on https://colab.research.google.com/drive/1WF6ui7Bk4x9PjRZm5tGM5F1DMBx22Jkt?usp=sharing
and last step, I trying convert language to Thai doctor on
https://colab.research.google.com/drive/1VvLfoiKguIJYZ65VBpMXJ-AhU5Spv1dT?usp=sharing
Thank you.